Java
This page contains all Java blog posts which include general java articles, java tutorials, java how-to articles as well as java snippets, examples, and GitHub Gists catalog. It also includes articles and tutorials on java supporting frameworks like hibernate (ORM) and Maven.
Wishing happy learning to all java beginners and geeks!

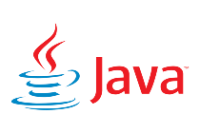

JAVA | ALL
JAVA | HOW-TOs
JAVA | TUTORIALS
JAVA CLASSES
HIBERNATE
MAVEN
JAVA GISTS
JAVA POSTS
Concatenate Strings Using String.join()
Java 8 has added a static join method to the String class. This concatenates Strings using a delimiter. Performance wise, it is similar to StringBuffer.append, but it has the added advantage that it automatically adds a delimiter. As before, this code creates string1 and string2. It invokes the join...
Concatenate Strings Using StringBuilder.append()
In addition to String, Java has the java.lang.StringBuffer and java.lang.StringBuilder classes. These can also be used to concatenate Strings. The advantage of these classes is that both these classes are mutable, so using them does not result in the creation of new String objects. So StringBuffer and StringBuilder...
Concatenate Strings Using StringBuffer.append()
In addition to String, Java has the java.lang.StringBuffer and java.lang.StringBuilder classes. These can also be used to concatenate Strings. The advantage of these classes is that both these classes are mutable, so using them does not result in the creation of new String objects. So StringBuffer and StringBuilder...
Concatenate Strings Using String.concat()
The String class has a method called concat. It accepts as parameter a String value and concatenates it with the current String. The following code demonstrates this: As before, this code creates string1 and string2. It then invokes the concat() method on string1 and passes string2 as an argument....
- Advertisement -
Concatenate Strings Using Plus Operator
In Java, a String is a sequence of characters. There are often programming situations where you will need to concatenate Strings. There are several ways in which you can achieve this. The + operator is also known as the concatenation operator. It can be used to concatenate Strings. The...
Remove Duplicates from List Using Stream
Java 8 has added the Stream API that helps to easily perform bulk operations on Collections. A new method called stream() method has been added to all the collection interfaces that returns a Stream corresponding to the underlying collection. The Stream interface has a method called distinct that can...
Java Tutorial #2 – Operators in Java
Table of Contents Introduction 1. Assignment Operator 2. Arithmetic Operators 3. Compound Operators 4. Increment & Decrement Operators 5. Relational Operators 6. Logical Operators Introduction Operators are an essential part of any programming language. In-fact it is not possible not to write a decent piece of code without making use of 'Operators'. Java operators...
Convert List to Array Using ToArray With Param
There is an overloaded version of the toArray method. This accepts an array as a parameter and returns a result array that is of the same data type as the input array. So basically, this toArray method can be used to obtain an array that is of the same data type...
- Advertisement -
Remove Duplicates from List Using Set.addAll
The Set interface has a method called addAll. This accepts a 'Collection' as the parameter. So if you invoke this method by passing the 'List', the duplicates will be eliminated. The following code snippet demonstrates this method. Here, a new LinkedHashSet is created and the addAll method is invoked by...
How To Remove Duplicates From List in Java
Introduction A List is an interface in the Java collection framework which can be used to store a group of objects. A List is ordered and it allows duplicates. However, there are often scenarios where you would need to eliminate duplicate elements from a List. There are several ways you can...
Convert List to Array Using ToArray Without Param
Java provides a toArray method on the 'List' interface. This can be used to convert a 'List' to an array. Since there are are two overloaded versions, one of these method is explained below. This toArray method does not accept any input parameters. It returns an object array. The following...
POPULAR | TAGS
LATEST POSTS
TOP | CATEGORIES
LATEST | JAVA GISTS
Rounding Decimal Number With Java DecimalFormat
The DecimalFormat class has a method called setRoundingMode() which can be used for setting the rounding mode for DecimalFormat object. The setRoundingMode() accepts RoundingMode...
Format Decimal Numbers with Grouping Separator
The DecimalFormat class has a method called setGroupingSize() which sets how many digits of the integer part to group. Groups are separated by the...
Format Decimal Numbers using Strings Within Pattern
As mentioned in earlier posts, the java.text.DecimalFormat class is used to format decimal numbers via predefined patterns specified as String. Apart from the decimal separator,...